In this example we will create a temperature meter using a mcp9808 sensor and display the readings on an LCD shield
Lets look at the mcp9808 first
The MCP9808 digital temperature sensor converts temperatures between -20°C and +100°C to a digital word with ±0.5°C (max.) accuracy. The MCP9808 comes with user-programmable registers that provide flexibility for temperature sensing applications.
The registers allow user-selectable settings such as Shutdown or low-power modes and the specification of temperature Event and Critical output boundaries. When the temperature changes beyond the specified boundary limits, the MCP9808 outputs an Event signal.
The user has the option of setting the event output signal polarity as an active-low or active-high comparator output for thermostat operation, or as temperature event interrupt output for microprocessor-based systems. The event output can also be configured as a Critical temperature output.
This sensor has an industry standard 2-wire, SMBus and Standard I2C™Compatible compatible (100kHz/400kHz bus clock) serial interface, allowing up to eight sensors to be controlled in a single serial bus.
Features
Accuracy:
±0.25°C (typical) from -40°C to +125°C
±0.5°C (maximum) from -20°C to +100°C
User Selectable Measurement Resolution:
0.5°C, 0.25°C, 0.125°C, 0.0625°C
User Programmable Temperature Limits:
Temperature Window Limit
Critical Temperature Limit
User Programmable Temperature Alert Output
Operating Voltage Range: 2.7V to 5.5V
More details about the sensor at http://www.microchip.com/wwwproducts/en/MCP9808
Connection
An easy sensor to connect which we connect to the LCD keypad shield like this
Parts List
Connection
In this example we show the connections to a typical LCD shield
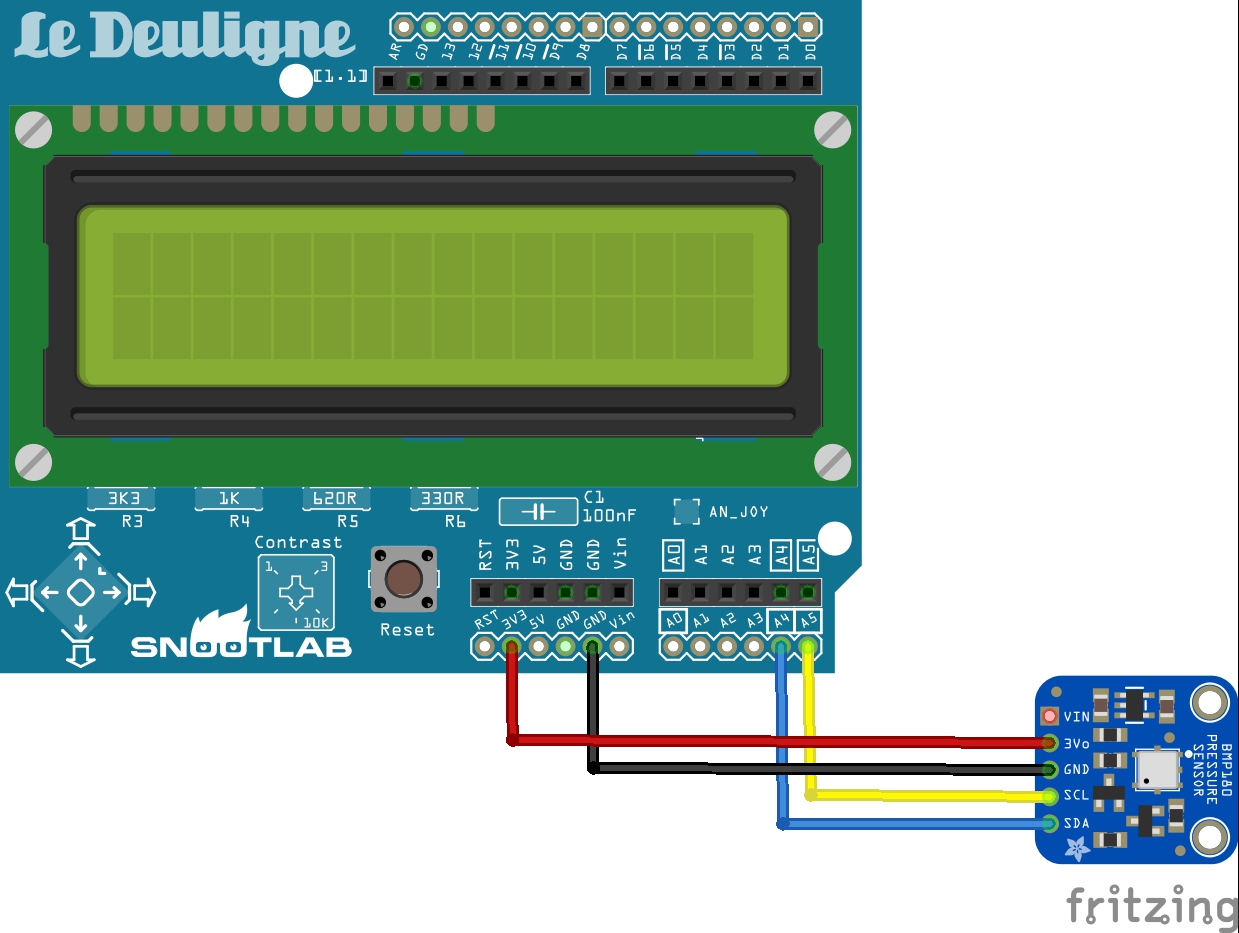
lcd shield and bmp180
Code
You need to add the adafruit mcp9808 library, this is available in the library manager
[codesyntax lang=”cpp”]
#include <Wire.h>
#include <LiquidCrystal.h>
#include “Adafruit_MCP9808.h”
//setup for the LCD keypad shield
LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
Adafruit_MCP9808 tempsensor = Adafruit_MCP9808();
void setup()
{
//init serial for some debug
Serial.begin(9600);
Serial.println(“MCP9808 Sensor Test”);
//init MCP9808
if (!tempsensor.begin())
{
Serial.println(“Couldn’t find MCP9808!”);
while (1);
}
//init LCD
lcd.begin(16,2);
//line 1 – temperature
lcd.setCursor(0,0);
lcd.print(“Temp = “);
}
void loop()
{
//read temperature in
float c = tempsensor.readTempC();
//convert to fahrenheit – not used in this example
float f = c * 9.0 / 5.0 + 32;
//display temperature and altitude on lcd
Serial.print(“Temp: “); //debug
Serial.println(c); //debug
lcd.setCursor(0,0);
lcd.print(“Temp = “);
lcd.print(c);
delay(1000);
}
[/codesyntax]